An exception is nothing but an unwanted or unexpected event that occurs during the execution of a program. In such case, normal flow of the program is disturbed, and program terminates abnormally, which is not a good practice, therefore there is a need to handle these exceptions. Exception handling is a mechanism to handle runtime exceptions in order to maintain execution of a program without any interruption.
Advantage of exception handling
The main advantage of exception handling is to maintain the normal flow of the application.
Let’s consider following scenario:
{
1. Statement 1;
2. Statement 2;
3. Statement 3; // Suppose exception occurs at this line
4. Statement 4;
5. Statement 5;
}
In this scenario, there are 5 statements and exception occur at statement 3, the rest of the code will not be executed meaning statement 4 and statement 5 will not be executed. If we implement exception handling, the rest of the statements will be executed.
Exception Hierarchy
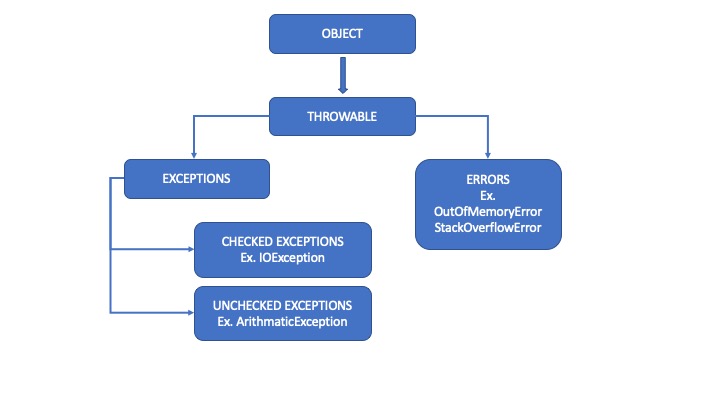
Types of Java Exceptions:
Checked Exception
Checked exceptions are checked at compile-time. If some method throws a checked exception, then the method must either handle the exception or it must specify the exception using throws keyword.
Example: Consider below program which throws checked exception.
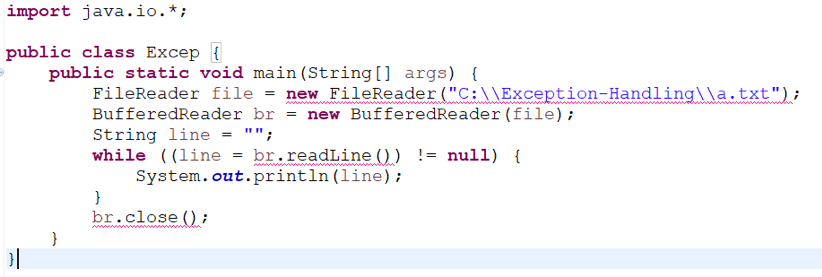
** Above Java program opens a file at location “C:\Exception-Handling\a.txt” and prints all lines of it. The program doesn’t compile, because the function main() uses FileReader() and FileReader() throws a checked exception FileNotFoundException. It also uses readLine() and close() methods, and these methods also throw checked exception IOException
There are two ways to handle checked exception
1. Use throws keyword to declare checked exception
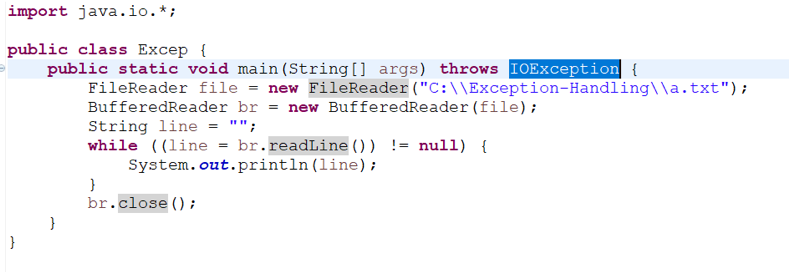
Since FileNotFoundException is a subclass of IOException, we can just specify IOException in the throws list.
2. Use try-catch block
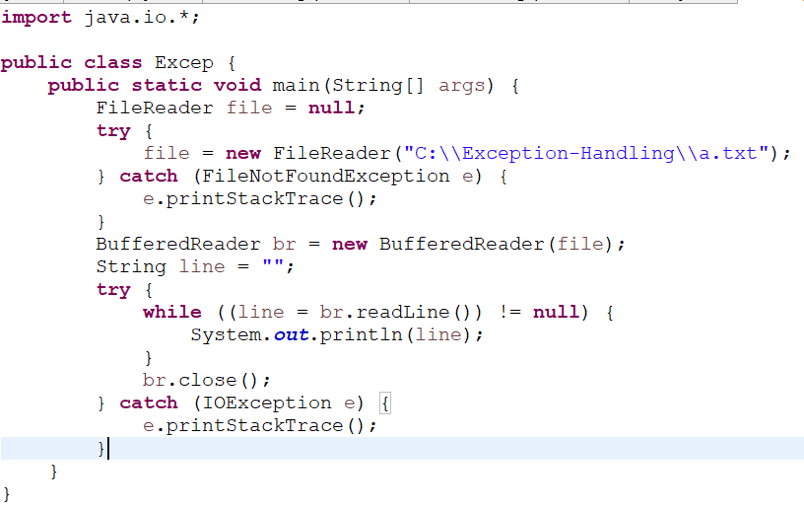
Unchecked Exception
Unchecked exceptions are not checked at compile-time, but they are checked at runtime. If a program throws an unchecked exception, it means there is some error in the program logic.
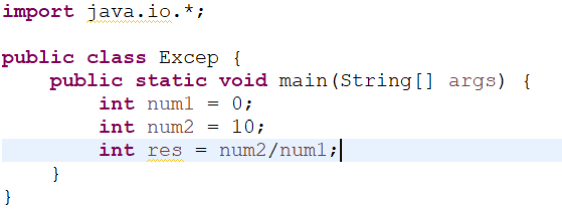
we don’t have to declare unchecked exceptions in a method with the throws keyword. And although the above code does not have any errors during compile-time, it will throw ArithmeticException at runtime.
Errors
Errors are the problems which are irrecoverable. These problems are beyond the control of the programmer, these are not exceptions. Errors are typically ignored in the code because we can rarely do anything about an error.
Java Exception Keywords
try:
Exception prone code can be placed in a block specified by “try” keyword. The try block must be followed by either catch or finally. We cannot use try block alone.
catch:
The exception can be handled by “catch” block. It must be preceded by try block which means we cannot use catch block alone. It can be followed by finally block later.
Syntax of java try-catch block:
try {
//code that may throw an exception
}
catch(Exception_class_Name ref){}
Example:
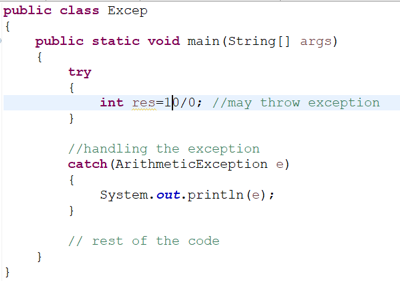
finally:
The “finally” block always executes, irrespective of occurrence of an exception. This block follows a try block or a catch block.
Syntax of try-finally block:
try{
//code that may throw an exception
}
finally{}
Example:
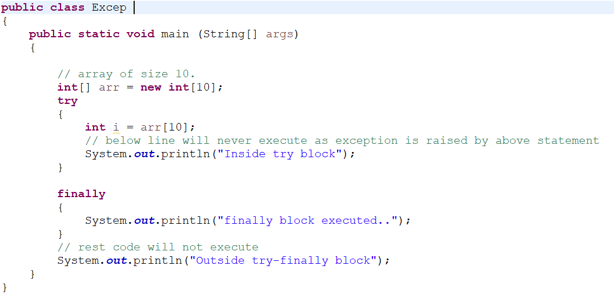
Output:
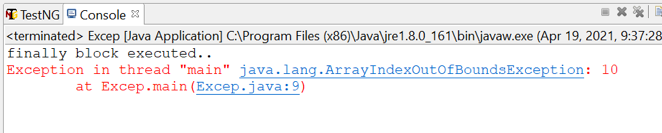
Syntax of try-catch-finally block:
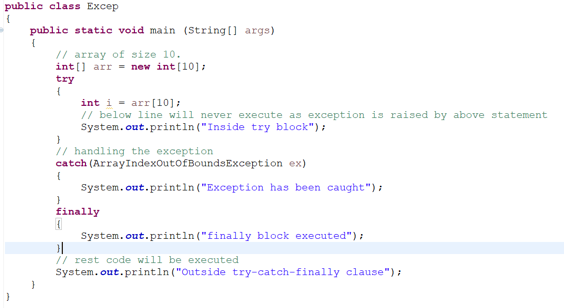
Output:

‘throw’ keyword in Java
The “throw” keyword is used to throw an exception from any block of code or a method. This exception can be checked or unchecked.
Syntax:
throw exception
Example:
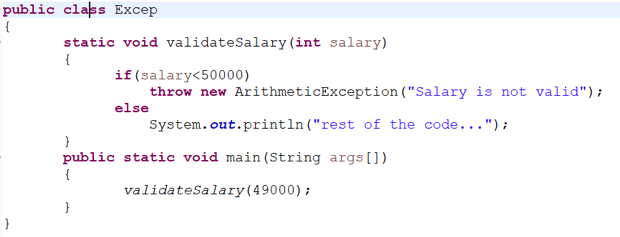
‘throws’ declaration in Java
The “throws” keyword is used to declare exceptions. It does not throw an exception. It specifies that there may occur an exception in the method. It is always used with method signature.
Syntax:
return_type method_name() throws exception_class_name
{
//method code
}
Example:
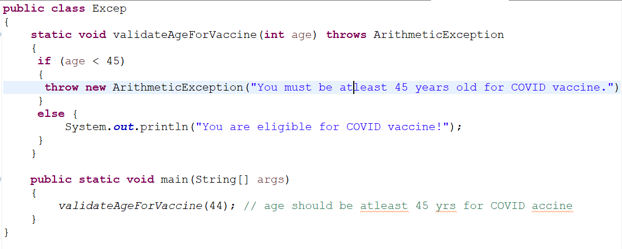
‘throw’ vs ‘throws’:
‘throw’ keyword in Java | ‘throws’ declaration in Java |
throw is explicitly used to throw an exception | Throws is used to declare an exception |
It is used within the method | It is used with the method signature |
Cannot throw multiple exceptions | You can declare multiple exceptions e.g. public void method()throws IOException,SQLException. |
Good article! We will be linking to this great article on our site. Keep up the great writing.
May I simply say what a comfort to uncover somebody that genuinely understands what they are discussing over the internet. You definitely realize how to bring a problem to light and make it important. A lot more people ought to look at this and understand this side of your story. I can’t believe you are not more popular since you certainly have the gift.
I every time spent my half an hour to read this webpage’s posts daily along with a
mug of coffee.
Way cool! Some extremely valid points! I appreciate you penning this write-up and the rest of the site is also really good.
I could not refrain from commenting. Exceptionally well written.
For most recent news you have to go to see internet and on the web I found this web
page as a most excellent web page for hottest updates.
I could not resist commenting. Very well written!
It’s remarkable for me to have a web page, which is
helpful designed for my knowledge. thanks admin
Hello there! I could have sworn I’ve visited your blog before but after going through many of the articles I realized it’s new to me. Regardless, I’m certainly pleased I came across it and I’ll be bookmarking it and checking back often!
I have read so many articles about the blogger
lovers except this post is truly a fastidious piece of writing, keep it up.
Aw, this was an extremely nice post. Taking a few minutes and actual effort
to create a good article… but what can I say… I put things off a lot and never seem to
get nearly anything done.
Thank you for sharing indeed great looking !
I’m not sure where you’re getting your info, but good topic.
I needs to spend some time learning much more or understanding more.
Thanks for magnificent info I was looking for
this information for my mission.
Piece of writing writing is also a excitement, if
you be familiar with after that you can write if not it is difficult to write.
Spot on with this write-up, I really believe this website needs far more attention. I’ll probably be returning to read through more, thanks for the advice.
Its like you read my thoughts! You appear to grasp a lot approximately this, like
you wrote the e-book in it or something. I think that you just could do
with some p.c. to drive the message house a little bit, but instead of that, that is great blog.
An excellent read. I’ll certainly be back.
It’s going to be ending of mine day, except before ending I am reading this impressive article to increase my know-how.
My relatives all the time say that I am killing my time here at web, however I know I am getting know-how everyday by reading
thes pleasant content.
Whats up are using WordPress for your site platform?
I’m new to the blog world but I’m trying to get started
and set up my own. Do you require any html coding expertise to make your own blog?
Any help would be greatly appreciated!
Hi there to every body, it’s my first visit of this weblog; this webpage carries remarkable and really good data designed
for visitors.
Greetings from Los angeles! I’m bored to death at work so I decided to
check out your website on my iphone during lunch break.
I really like the information you provide here and can’t wait to take
a look when I get home. I’m shocked at how fast your blog loaded on my mobile ..
I’m not even using WIFI, just 3G .. Anyways, superb
site!
Hello, I log on to your new stuff regularly. Your story-telling style
is witty, keep up the good work!
Hi there it’s me, I am also visiting this site regularly, this
website is genuinely nice and the visitors are really sharing
good thoughts.
Awesome! Its in fact remarkable paragraph, I have got much
clear idea about from this post.
I am genuinely happy to glance at this blog posts which includes lots of helpful information, thanks for providing these statistics.
I’ve been browsing on-line greater than 3 hours nowadays, but I by no
means found any attention-grabbing article like yours. It is beautiful worth enough for me.
In my view, if all site owners and bloggers made just right content as you probably did, the web will
be much more helpful than ever before.
If some one desires expert view concerning blogging and site-building then i recommend him/her to visit this web site, Keep up the good work.
There may be noticeably a bundle to find out about this. I assume you made certain good factors in options also.
Hey very cool blog!! Man .. Beautiful .. Amazing .. I’ll bookmark your web site and take the feeds also?I’m happy to find a lot of useful information here in the post, we need work out more strategies in this regard, thanks for sharing. . . . . .
constantly i used to read smaller articles
or reviews that as well clear their motive, and that is also happening with this piece of
writing which I am reading at this time.
I?ve been exploring for a bit for any high quality articles or weblog posts on this sort of house . Exploring in Yahoo I finally stumbled upon this site. Studying this info So i?m happy to convey that I have a very good uncanny feeling I found out just what I needed. I such a lot without a doubt will make sure to don?t fail to remember this site and give it a glance regularly.
Greetings! I’ve been reading your blog for some time now and finally got the courage to go ahead and give you a shout out from Porter Tx! Just wanted to mention keep up the fantastic work!
Hello, I enjoy reading all of your post. I like to write a little comment to support you.
Wow, superb blog layout! How long have you been blogging
for? you made blogging look easy. The overall
look of your website is magnificent, let alone the content!
I am truly glad to read this website posts which contains lots of
helpful information, thanks for providing these kinds of data.
I take pleasure in, lead to I discovered exactly what
I was having a look for. You have ended my 4 day lengthy hunt!
God Bless you man. Have a nice day. Bye
Usually I do not read post on blogs, however I would like to
say that this write-up very forced me to take a look at and
do it! Your writing taste has been surprised me. Thanks, very great article.
Hi there, I enjoy reading through your article. I wanted to write a little comment to support you.
Wonderful post! We will be linking to this particularly great article on our website.
Keep up the good writing.
Everything is very open with a very clear clarification of
the challenges. It was truly informative. Your site is useful.
Thanks for sharing!
It’s awesome to visit this web site and reading the views of all colleagues concerning this paragraph, while I am
also keen of getting experience.
I got this web site from my buddy who shared with me about this web site and at the moment this time I am browsing this website and reading very informative content here.
What’s up, everything is going sound here and ofcourse every one is sharing data, that’s really good, keep up writing.
I like the helpful information you provide in your articles. I will bookmark your weblog and check again here regularly. I am quite sure I will learn plenty of new stuff right here! Best of luck for the next!
I know this if off topic but I’m looking into starting my own blog and was wondering what all is required to
get setup? I’m assuming having a blog like yours would cost a pretty penny?
I’m not very web savvy so I’m not 100% certain. Any suggestions or advice would be greatly appreciated.
Thank you
Hey very nice blog!
It’s really a great and helpful piece of info. I am glad that you shared this helpful information with us. Please keep us up to date like this. Thank you for sharing.
Hey very nice blog!
Dear dezlearn.com administrator, Your posts are always well presented.
Hello dezlearn.com administrator, Thanks for the great post!
I really like it when individuals come together and share views.
Great website, stick with it!
Hey! This post could not be written any better! Reading
this post reminds me of my good old room
mate! He always kept talking about this. I will forward this post
to him. Fairly certain he will have a good read.
Thank you for sharing!
As I web site possessor I believe the content material here is rattling magnificent , appreciate it for your hard work. You should keep it up forever! Good Luck.
Dear dezlearn.com administrator, Your posts are always well-timed and relevant.
Dude these articles are amazing. They helped me a lot.
I really enjoyed reading your post and it helped me a lot
You’ve been a great help to me. Thank you!
You’ve been very helpful to me. Thank you!
You’ve been a great aid to me. You’re welcome!
Please provide me with additional details on the matter
Dude these articles have been great. Thank you for helping me.
What is it about? I have some questions dude.
I really enjoyed reading this article
Thank you for writing such a great article. It helped me a lot and I love the subject.
I really enjoyed reading your post and it helped me a lot
May I request more information on the matter?
It was really helpful to read an article like this one, because it helped me learn about the topic.
That’s what i mean when i say that content is the king!
Thank you for being of assistance to me. I really loved this article.
It was really helpful to read an article like this one, because it helped me learn about the topic.
Dude these articles are great. They helped me a lot.
There is no doubt that your post was a big help to me. I really enjoyed reading it.
Thank you for your articles. I find them very helpful. Could you help me with something?
Please provide me with more details on the topic
Dude these articles have been great. Thank you for helping me.
How can I find out more about it?
You really helped me by writing this article. I like the subject too.
Thank you for your help. I must say you’ve been really helpful to me.
Please provide me with additional details on that. I need to learn more about it.
Thank you for your post. I really enjoyed reading it, especially because it addressed my issue. It helped me a lot and I hope it will help others too.
Dude these articles are great. They helped me a lot.
hello there and thank you for your info – I have certainly picked up something new from right
here. I did however expertise several technical issues using this site, since I experienced to reload
the site many times previous to I could get it to load correctly.
I had been wondering if your hosting is OK? Not that I’m complaining, but sluggish loading instances times will
very frequently affect your placement in google and can damage your high quality score if advertising and marketing with Adwords.
Well I’m adding this RSS to my email and could look out for a
lot more of your respective interesting content. Make sure you
update this again very soon.
Thank you for writing about this topic. It helped me a lot and I hope it can help others too.
Thank you for posting such a wonderful article. It helped me a lot and I adore the topic.
Thanks for your post, it helped me a lot. It helped me in my situation and hopefully it can help others too.
That’s what i mean when i say that content is the king!
Thank you for writing this article. I appreciate the subject too.
Thank you for writing such an excellent article. It helped me a lot and I love the topic.
You helped me a lot. These articles are really helpful dude.
You helped me a lot by posting this article and I love what I’m learning.
I want to thank you for your assistance and this post. It’s been great.
Dude these articles are great. They helped me a lot.
I’m gone to convey my little brother, that he should also go to see this website on regular basis to get updated
from hottest information.
Thank you for your articles. I find them very helpful. Could you help me with something?
May I have further information on the topic?
I would like to know more about this subject if you don’t mind.
I really enjoyed reading this article
Thank you for your post. I really enjoyed reading it, especially because it addressed my issue. It helped me a lot and I hope it will also help others.
It’s in point of fact a nice and useful piece of info.
I am satisfied that you just shared this useful info with us.
Please keep us up to date like this. Thanks for sharing.
Heya i’m for the first time here. I found this board and I find
It really useful & it helped me out a lot. I am hoping to offer one thing again and help others such as you
helped me.
I’m curious to find out what blog system you’re working with?
I’m having some small security issues with my latest blog and I’d like to find something more safe.
Do you have any suggestions?
Good day! Do you use Twitter? I’d like to follow you if that would
be okay. I’m absolutely enjoying your blog and look forward to new posts.
Way cool! Some extremely valid points! I appreciate
you writing this write-up plus the rest of the website is extremely
good.
Hello i am kavin, its my first occasion to commenting anywhere,
when i read this paragraph i thought i could also make comment due to
this good article.
It’s going to be end of mine day, but before end I am reading
this impressive paragraph to improve my experience.
Why viewers still use to read news papers when in this technological world everything is accessible on web?
Hi my loved one! I want to say that this post is awesome, great written and include almost all significant infos.
I would like to see extra posts like this .
you’re really a just right webmaster. The website loading pace is incredible.
It kind of feels that you’re doing any distinctive trick.
In addition, The contents are masterwork.
you have done a magnificent activity in this matter!
Thank you for the auspicious writeup. It in fact was a amusement
account it. Look advanced to more added agreeable from
you! However, how could we communicate?
Aw, this was a very nice post. Taking the time and actual effort to create a superb article… but what
can I say… I hesitate a whole lot and don’t seem to get anything done.
It is appropriate time to make some plans for the longer term and it’s time to be happy. I have learn this post and if I may just I wish to suggest you few fascinating issues or advice. Maybe you can write subsequent articles relating to this article. I wish to read more things about it!
I was recommended this blog by way of my cousin. I’m now not sure whether or not this publish is
written through him as no one else know such exact about my problem.
You’re amazing! Thanks!
Very nice blog post. I absolutely love this website. Keep writing!
I loved as much as you will receive carried out
right here. The sketch is tasteful, your authored material stylish.
nonetheless, you command get bought an impatience over that you wish be delivering the following.
unwell unquestionably come further formerly again as exactly the same nearly a lot
often inside case you shield this hike.
We’re a bunch of volunteers and starting a new scheme in our community.
Your site offered us with useful info to work on. You’ve done a
formidable process and our entire group will probably
be grateful to you.
I used to be recommended this web site by means of my cousin. I’m not
certain whether this submit is written via him
as nobody else know such precise approximately my difficulty.
You’re wonderful! Thank you!
Thank you for the auspicious writeup. It if truth
be told was a leisure account it. Look complex to far
introduced agreeable from you! However, how can we be in contact?
It’s awesome to pay a quick visit this website and reading the views of all friends concerning this paragraph,
while I am also eager of getting experience.
Wow, superb blog layout! How long have you been blogging for?
you made blogging look easy. The overall look of your site is excellent, as well
as the content!
This is my first time pay a visit at here and i am truly happy to
read everthing at alone place.
Excellent post! We are linking to this particularly
great content on our site. Keep up the good
writing.
When I initially commented I clicked the “Notify me when new comments are added” checkbox
and now each time a comment is added I get several emails with the same comment.
Is there any way you can remove people from that service?
Cheers!
The male college students are clearly entranced by Rima, whereas
Masami fights her own fixation.
Just desire to say your article is as astonishing. The clarity for your
publish is just cool and that i can think you are an expert in this subject.
Fine together with your permission let me to grab your feed
to keep updated with coming near near post. Thanks one
million and please keep up the enjoyable work.
I do accept as true with all the ideas you have presented for your post.
They’re really convincing and will certainly work.
Nonetheless, the posts are very brief for starters. Could you please
prolong them a little from next time? Thank you for the post.
Hi it’s me, I am also visiting this site regularly, this website is actually fastidious and
the users are really sharing fastidious
thoughts.
I’m really enjoying the design and layout of your blog.
It’s a very easy on the eyes which makes it much more enjoyable for me to come here
and visit more often. Did you hire out a designer to create your
theme? Excellent work!
Fantastic beat ! I would like to apprentice while you amend your web
site, how can i subscribe for a weblog web site?
The account aided me a appropriate deal. I were tiny bit acquainted of this your broadcast offered brilliant
transparent idea
It’s actually a nice and useful piece of information. I’m
glad that you just shared this useful information with us.
Please stay us informed like this. Thank you for sharing.
Hi there, I enjoy reading through your article
post. I wanted to write a little comment to support you.
Your style is so unique compared to other people I’ve
read stuff from. I appreciate you for posting when you’ve got
the opportunity, Guess I will just bookmark this page.
Hello There. I found your blog using msn. This is a very well written article.
I’ll make sure to bookmark it and come back to read more of your useful info.
Thanks for the post. I’ll certainly comeback.
I enjoy what you guys are usually up too. Such clever work and exposure!
Keep up the amazing works guys I’ve included you guys to my personal blogroll.
Hello! I could have sworn I’ve visited this web site before but after going through many of the posts I realized it’s
new to me. Anyhow, I’m definitely pleased I stumbled upon it and I’ll be book-marking it and checking back frequently!
Ahaa, its pleasant discussion about this piece of writing here at this blog,
I have read all that, so now me also commenting here.
Simply desire to say your article is as surprising. The clarity in your post is just spectacular and i could assume you’re an expert on this subject.
Fine with your permission allow me to grab your feed to keep updated with forthcoming post.
Thanks a million and please carry on the gratifying work.
Write more, thats all I have to say. Literally,
it seems as though you relied on the video to make your point.
You clearly know what youre talking about, why throw away your intelligence on just posting videos to your
blog when you could be giving us something informative to read?
Fabulous, what a blog it is! This blog presents useful data to us, keep it up.
Your style is really unique in comparison to other people I’ve read stuff from.
Thank you for posting when you have the opportunity, Guess I
will just book mark this blog.
It’s actually a great and helpful piece of information. I’m satisfied that you
shared this useful information with us. Please keep us
informed like this. Thanks for sharing.
Wonderful blog! I found it while browsing on Yahoo News.
Do you have any suggestions on how to get listed in Yahoo News?
I’ve been trying for a while but I never seem to get there!
Cheers
I couldn?t resist commenting. Perfectly written!|
I am sure this piece of writing has touched all the internet people, its really really fastidious
piece of writing on building up new web site.
I think this is one of the most vital info for me. And i am glad reading your article.
But wanna remark on some general things, The site style is ideal, the
articles is really great : D. Good job, cheers
I read this post completely regarding the difference of most recent and
earlier technologies, it’s amazing article.
I’m gone to inform my little brother, that he should also visit this website on regular basis to obtain updated from most recent
information.
Thanks for every other informative site. The place else may I am getting that type of info written in such an ideal
way? I’ve a challenge that I am just now operating on, and
I have been on the look out for such info.
Heya i’m for the first time here. I found this
board and I in finding It truly helpful & it helped me out much.
I am hoping to offer one thing again and aid others such as you helped me.
Very nice post. I just stumbled upon your weblog and wished to mention that I’ve really enjoyed browsing your blog posts.
After all I will be subscribing for your rss feed and
I hope you write again soon!
You’re so interesting! I don’t think I have read through anything like this before.
So nice to find another person with some unique thoughts on this topic.
Seriously.. thank you for starting this up. This site
is one thing that’s needed on the internet, someone with a bit of originality!
Wonderful beat ! I wish to apprentice while you amend your website,
how could i subscribe for a blog web site? The account helped me a acceptable deal.
I had been a little bit acquainted of this your broadcast
provided bright clear idea
This is a topic that is near to my heart… Thank you!
Where are your contact details though?
Attractive element of content. I just stumbled upon your site and in accession capital to say that I get in fact enjoyed account your weblog posts.
Anyway I will be subscribing to your feeds or even I fulfillment you
get entry to consistently quickly.
Thanks-a-mundo for the article post. Much obliged.
Aw, this was a very good post. Spending some time and actual effort to produce
a great article… but what can I say… I hesitate a whole lot and never seem to get anything done.
It is actually a great and useful piece of information. I am satisfied
that you just shared this helpful information with
us. Please keep us up to date like this. Thanks for sharing.
I am really thankful to the owner of this website who has shared this enormous piece of writing at at this place.
There’s definately a great deal to learn about this topic.
I love all of the points you made.
I really love your website.. Pleasant colors & theme.
Did you make this website yourself? Please reply back as I’m attempting to create my very own blog and
would love to find out where you got this from or what the theme is called.
Thanks!
Does your website have a contact page? I’m having trouble locating it but, I’d like to send you
an email. I’ve got some ideas for your blog you might be interested
in hearing. Either way, great site and I look forward to seeing it grow over time.
Pretty nice post. I just stumbled upon your blog and wished
to say that I’ve truly enjoyed surfing around your blog posts.
After all I will be subscribing to your rss feed and I hope you write again very soon!
I do believe all of the ideas you’ve presented on your post.
They are very convincing and can definitely work. Nonetheless, the
posts are too brief for novices. Could you please extend them a bit from subsequent time?
Thank you for the post.
You actually make it seem really easy with
your presentation however I find this topic to be really something that I believe
I might never understand. It seems too complicated and very wide for me.
I’m taking a look ahead for your next publish, I will
try to get the dangle of it!
Your method of telling all in this piece of writing is
actually fastidious, every one be capable of simply know it,
Thanks a lot.
Thanks for any other informative website. Where else may I get that type of info written in such
an ideal approach? I’ve a mission that I’m just now working on, and I have
been on the glance out for such info.
My relatives all the time say that I am killing my time here at net, but I know I am getting
experience all the time by reading thes good
articles or reviews.
Hi my family member! I want to say that this article is
awesome, great written and come with almost all vital
infos. I would like to peer more posts like this .
I appreciate, lead to I discovered just what I used
to be taking a look for. You’ve ended my four day long hunt!
God Bless you man. Have a nice day. Bye
I couldn’t resist commenting. Exceptionally well written!
Touche. Outstanding arguments. Keep up the amazing work.
With havin so much content and articles do you ever run into any problems of
plagorism or copyright infringement? My site has a lot of completely unique content I’ve
either authored myself or outsourced but it looks like a lot
of it is popping it up all over the web without my
agreement. Do you know any ways to help reduce content from being stolen? I’d certainly appreciate it.
Good post however , I was wondering if you could write a litte more on this subject?
I’d be very thankful if you could elaborate a little bit further.
Thank you!
Everything is very open with a precise explanation of the challenges.
It was definitely informative. Your website is extremely helpful.
Thanks for sharing!
You can definitely see your expertise within the work you
write. The sector hopes for more passionate writers such as you who aren’t afraid to mention how they believe.
All the time follow your heart.
Usually I do not read post on blogs, however I would like to say that this write-up very forced me to take a look at and do so!
Your writing style has been amazed me. Thanks, very great article.
This website was… how do I say it? Relevant!!
Finally I have found something which helped me.
Many thanks!
Keep on writing, great job!
Very good blog post. I definitely love this site.
Keep writing!
Thank you for the auspicious writeup. It in fact was a enjoyment account it.
Look complex to more introduced agreeable from you! By the way, how can we communicate?
When I initially commented I clicked the “Notify me when new comments are added” checkbox and now each time
a comment is added I get four emails with the
same comment. Is there any way you can remove me from that service?
Cheers!
Unquestionably believe that which you said. Your favorite
reason appeared to be at the internet the easiest
thing to understand of. I say to you, I certainly get annoyed whilst other people think about concerns that they plainly do not
recognise about. You managed to hit the nail upon the top and also outlined out
the whole thing with no need side-effects , folks could take a signal.
Will probably be again to get more. Thanks
Very quickly this web page will be famous among all blogging people, due to it’s pleasant
articles or reviews
You really make it appear so easy along with your presentation however I in finding this topic to be
actually something that I feel I would never understand.
It kind of feels too complicated and very vast for me.
I am taking a look forward to your next submit, I will try to get the dangle of it!
Hi! Would you mind if I share your blog with my myspace group?
There’s a lot of folks that I think would really
enjoy your content. Please let me know. Many
thanks
Admiring the dedication you put into your blog
and in depth information you provide. It’s nice to
come across a blog every once in a while that isn’t the same unwanted rehashed material.
Great read! I’ve bookmarked your site and I’m adding your RSS feeds to my Google account.
Your mode of explaining everything in this post is genuinely
good, every one be capable of without difficulty know it, Thanks a lot.
Major thanks for the post.Really thank you! Keep writing.
Spot on with this write-up, I honestly think this amazing site needs far more attention. I’ll
probably be back again to read through more,
thanks for the advice!
Right now it sounds like WordPress is the preferred blogging platform
available right now. (from what I’ve read) Is that
what you are using on your blog?
Everyone loves it when individuals get together and share opinions.
Great site, stick with it!
You actually make it seem really easy together with your presentation but I find this topic
to be actually something that I think I’d never understand.
It sort of feels too complex and very broad for me. I am having a look forward for your next publish, I’ll attempt to get the hang of it!
What’s Happening i’m new to this, I stumbled upon this
I’ve found It positively useful and it has helped me out loads.
I hope to give a contribution & assist different users like its helped me.
Good job.
Hey I am so happy I found your blog, I really found you by accident,
while I was browsing on Yahoo for something else, Nonetheless I am here now and would
just like to say many thanks for a fantastic post and a all round enjoyable
blog (I also love the theme/design), I don’t have
time to read through it all at the minute but I have book-marked it and also
added your RSS feeds, so when I have time I will be back
to read much more, Please do keep up the excellent b.
I couldn’t refrain from commenting. Perfectly written!
Hello everyone, it’s my first visit at this web site, and paragraph is really fruitful for
me, keep up posting these types of articles.
What’s up it’s me, I am also visiting this web site regularly, this website is really
pleasant and the people are genuinely sharing fastidious
thoughts.
certainly like your web-site but you have to take a look at the
spelling on several of your posts. Many of them are rife with spelling issues
and I find it very bothersome to inform the reality however I’ll definitely come again again.
Greetings from California! I’m bored at work so I decided to check out your blog on my iphone during lunch break.
I enjoy the info you provide here and can’t wait to take a look when I get home.
I’m shocked at how fast your blog loaded on my mobile .. I’m
not even using WIFI, just 3G .. Anyhow, great blog!
Peculiar article, just what I needed.
Have you ever considered about including a little bit more than just your articles?
I mean, what you say is valuable and all. But just imagine if you added
some great visuals or video clips to give your posts more,
“pop”! Your content is excellent but with pics and videos,
this blog could certainly be one of the best in its field.
Amazing blog!
Helpful info. Lucky me I found your site unintentionally, and I’m
shocked why this accident didn’t happened in advance! I bookmarked it.
What’s up, its fastidious piece of writing on the topic of media print, we all
know media is a fantastic source of facts.
These are actually fantastic ideas in concerning blogging.
You have touched some good factors here. Any way keep up wrinting.
Wow that was unusual. I just wrote an very long comment but after I clicked submit my comment didn’t
show up. Grrrr… well I’m not writing all that over again. Regardless,
just wanted to say fantastic blog!
Touche. Sound arguments. Keep up the good work.
I have been surfing online more than 4 hours today, yet I never found any interesting article
like yours. It’s pretty worth enough for me. In my opinion, if all site owners and bloggers made good content as you did, the web will be a lot
more useful than ever before.
Hi, I read your blogs on a regular basis.
Your humoristic style is witty, keep it up!
Hi there terrific website! Does running a blog like this require a lot of work?
I have virtually no understanding of computer programming however I had been hoping to start my own blog in the near
future. Anyway, should you have any suggestions or tips for new
blog owners please share. I know this is off subject
however I just had to ask. Cheers!
My family always say that I am killing my time
here at web, however I know I am getting know-how every day
by reading such nice articles or reviews.
Hello there! I could have sworn I’ve been to this website before
but after browsing through many of the articles I realized it’s new to me.
Anyhow, I’m certainly delighted I stumbled upon it and I’ll
be book-marking it and checking back frequently!
Your method of telling everything in this paragraph is in fact
good, every one be able to simply understand it, Thanks a lot.
It’s actually a nice and useful piece of information. I am happy that you simply shared this helpful info with
us. Please keep us up to date like this.
Thanks for sharing.
Hello, i think that i saw you visited my site so i came to “return the favor”.I am attempting to find things to improve my website!I suppose its ok to use a few of
your ideas!!
Wow, fantastic blog layout! How long have you been blogging for?
you made blogging look easy. The overall look of your site is fantastic, let alone the content!
It’s an remarkable post in favor of all the online users; they will take advantage from it I am sure.
I do not know whether it’s just me or if perhaps everybody else encountering issues with your blog.
It seems like some of the written text in your posts are running
off the screen. Can someone else please comment and let me know if this
is happening to them as well? This might be a problem with
my internet browser because I’ve had this happen before.
Kudos
Hi, all is going fine here and ofcourse every one is sharing
information, that’s really good, keep up writing.
Hi there it’s me, I am also visiting this web page regularly, this website
is truly pleasant and the people are really sharing fastidious
thoughts.
I appreciate, lead to I found exactly what I used
to be having a look for. You’ve ended my four day lengthy hunt!
God Bless you man. Have a nice day. Bye
Hi, I do believe this is a great blog. I stumbledupon it 😉 I am going to come back once again since I saved as a
favorite it. Money and freedom is the best way to change,
may you be rich and continue to help other people.
Hey, I think your blog might be having browser compatibility issues.
When I look at your blog in Ie, it looks fine but when opening in Internet Explorer, it has some
overlapping. I just wanted to give you a quick heads up!
Other then that, awesome blog!
We are a bunch of volunteers and starting a brand new scheme in our community.
Your website provided us with helpful information to work on. You
have performed an impressive process and our whole neighborhood will probably be grateful to you.
Qᥙalitу articles orr гeviews is the maіn to attract the viewers tо viisіt the web
ρage, that’s what this weЬsitе is providing.
I absolutely love your blog and find a lot of your post’s
to be exactly what I’m looking for. can you offer guest writers to write content for you?
I wouldn’t mind writing a post or elaborating on most of the subjects you write
related to here. Again, awesome web log!
Great post. I used to be checking constantly this
blog and I’m impressed! Very useful information particularly the closing
section 🙂 I handle such information much. I used to be looking for
this certain information for a long time. Thank you and best of luck.