Optional was introduced in Java 8 and its primary objective is to prevent NullPointer exceptions in code.
Consider it as wrapper/container object which may or may not hold a non-null value. One needs to unwrap/de-containerize it to get the actual content using methods provided by Optional itself. For example – method ‘isPresent‘ invoked on Optional instance returns true if it contains a non-null value and method ‘get’ will return the actual content.
Let us try to understand this with below example.
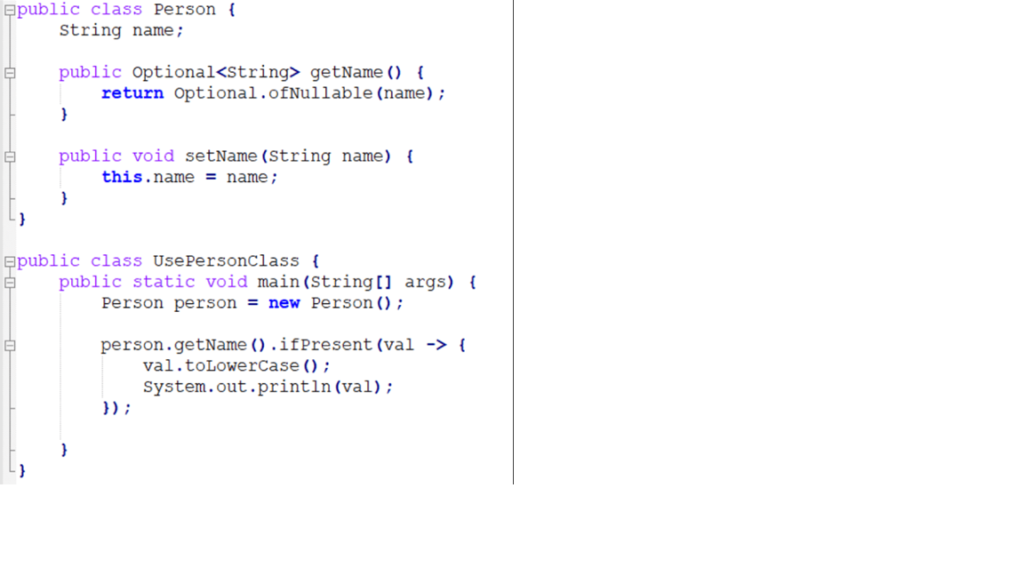
In class ‘UsePersonClass’ we use a zero-argument constructor to create instance of ‘Person’ class (line 17). Thus, member variable of the instance will hold null.
So ‘person.getName()’ on line 20, will return Null. And since we have called ‘toLowerCase’ method on Null object, it throws NullPointer exception.
If we analyse, the reason for this exception are-
- In ‘Person’ class we declared return type of ‘getName’ function as String. This does not mandate the consumer of this class (in this example, UsePersonClass is its consumer) to do a check for Null values.
- The consumer class, ‘UsePersonClass’ missed to put explicit condition to check value returned by ‘getName()’ before invoking further methods on it.
As is evident, putting a null check condition in above program, should have prevented any exception situation. But in large programs where there are multiple provider/model classes (like the ‘Person’ class in this example) the consumer class (like UsePersonClass’ class in this example), one will require to put null checks at multiple steps, which would make the program bulky and difficult to read.
Now let’s see how Optional helps in improving this situation by modifying the earlier provider and consumer classes.
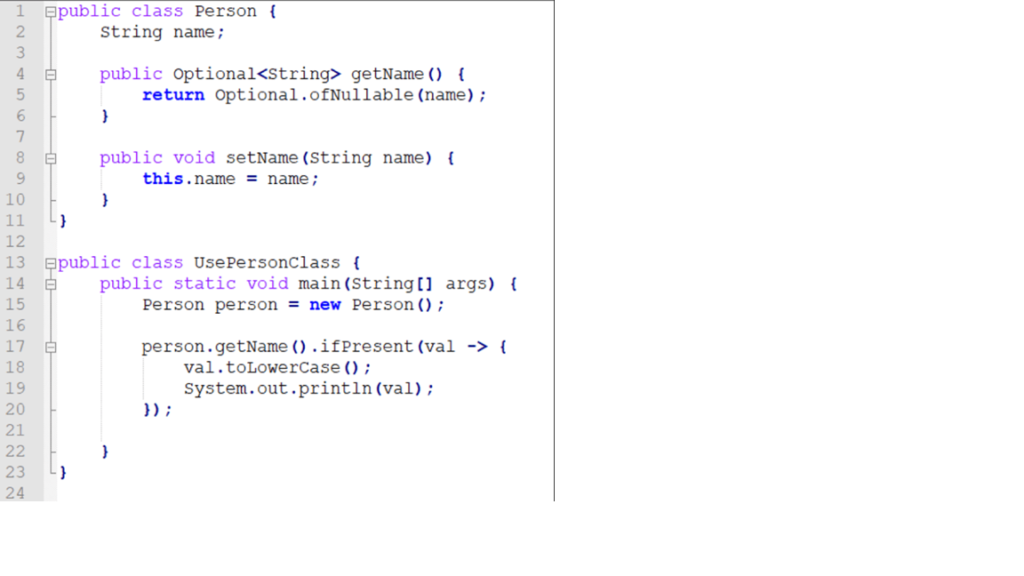
Highlights of this approach
- We have changed the return type of function ‘getName’ in ‘Person’ class, to return ‘Optional’ type rather than ‘String’ type (line 4)
- ‘Optional.ofNullable(name) converts the String value to an ‘Optional’ object.
- Now ‘UsePersonClass’ class CANNOT directly call ‘toLowerCase’ method as it is not available in ‘Optional’ type. Optional here forces user to first check if a non-null value is returned and only then perform further actions.
- There are other methods provided by Optional to verify, extract and process Optional object, in functional programming fashion.
Hence using Optional in Person class, we are hinting its consumer that it may or may not return a null value so the consumer can program accordingly and avoid Null Pointer exception.
Other ways to create instance of Optional
1. Optional<Object> personWrappedIntoOptional = Optional.of(person);
Use this only when you are sure that ‘person’ will not be Null. If there is possibility of ‘person’ to be Null, use Optional.ofNullable
2. Optional<Object> emptyOptional = Optional.empty();
Creates an empty Optional instance.
Illustration of usage of few methods provided by Optional:
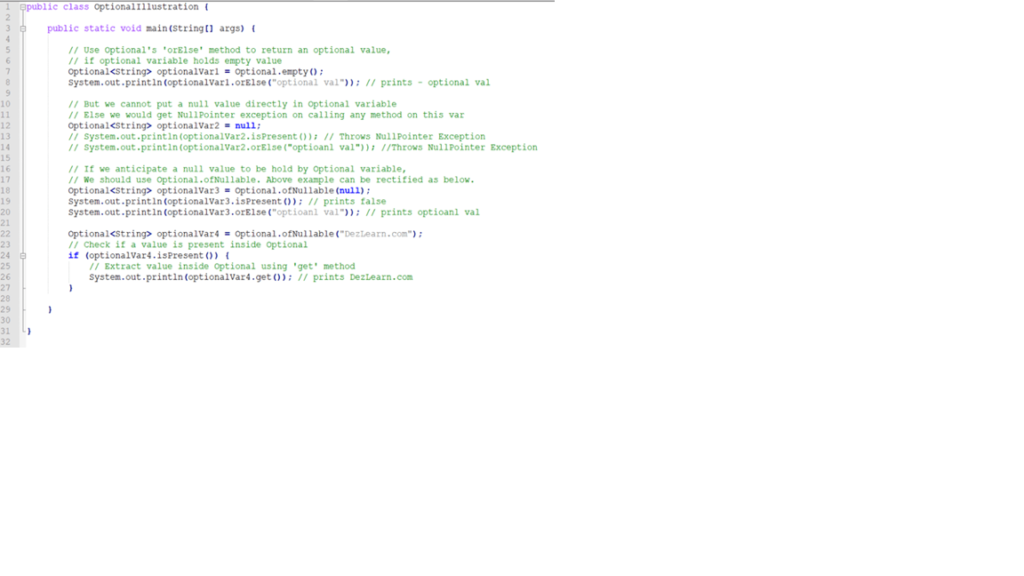
With havin so much written content do you ever run into any issues of plagorism or copyright violation? My site has a lot of completely unique content I’ve either created myself or outsourced but it looks like a lot of it is popping it up all over the web without my permission. Do you know any ways to help stop content from being stolen? I’d definitely appreciate it.
WOW just what I was searching for. Came here by searching for website
Simply want to say your article is as astonishing. The clarity in your post is just excellent and i could assume you are an expert on this subject. Fine with your permission allow me to grab your feed to keep updated with forthcoming post. Thanks a million and please keep up the enjoyable work.
Thank you for posting this. I really enjoyed reading it, especially because it addressed my question. It helped me a lot and I hope it will help others too.
I would like to know more about this subject if you don’t mind.
Please answer my question. How can i contact you regards this article?
I have to thank you for this article
Thank you for writing such a great article. It helped me a lot and I love the subject.
Thank you for writing about this topic. It helped me a lot and I hope it can help others too.
Dear can you please write more on this? Your posts are always helpful to me. Thank you!
Please tell me more about your excellent articles
You’ve been a great help to me. Thank you!
Wow! This can be one particular of the most useful blogs We’ve ever arrive across on this subject. Basically Wonderful. I am also a specialist in this topic so I can understand your effort.
Your house is valueble for me. Thanks!?
Please provide me with additional details on that. I need to learn more about it.
I must say you’ve been a big help to me. Thanks!
Dude these articles are great. They helped me a lot.
May I request more information on the matter?
I always find your articles very helpful. Thank you!
I really enjoyed reading your post and it helped me a lot
You helped me a lot. These articles are really helpful dude.
Dude these articles are great. They helped me a lot.
I always find your articles very helpful. Thank you!
Please provide me with additional details on the matter
I really enjoyed reading your post, especially because it addressed my issue. It helped me a lot and I hope it can help others too.
Thank you for your excellent articles. May I ask for more information?
I really enjoyed reading your post and it helped me a lot
You’ve been really helpful to me. Thank you!
Please tell me more about this
Thanks for posting. I really enjoyed reading it, especially because it addressed my issue. It helped me a lot and I hope it will help others too.
Your articles are extremely helpful to me. Please provide more information!
You should write more articles like this, you really helped me and I love the subject.
Your articles are very helpful to me. May I request more information?
I really enjoyed reading your post and it helped me a lot
Thank you for your excellent articles. Would you be able to help me out?
Thank you for your articles. I find them very helpful. Could you help me with something?
Dude these articles are great. They helped me a lot.
Thank you for posting such a wonderful article. It really helped me and I love the topic.
Thank you for posting this. I really enjoyed reading it, especially because it addressed my question. It helped me a lot and I hope it will help others too.
I really enjoyed reading your post, especially because it addressed my issue. It helped me a lot and I hope it can help others too.
Please answer my question. How can i contact you regards this article?
Thank you for writing this article. I appreciate the subject too.
Thank you for your articles. I find them very helpful. Could you help me with something?
May I request more information on the subject? All of your articles are extremely useful to me. Thank you!
Thank you for writing about this topic. Your post really helped me and I hope it can help others too.
You helped me a lot. These articles are really helpful dude.
I really enjoyed reading this article
You’ve been terrific to me. Thank you!
Thank you for writing this article!
I always find your articles very helpful. Thank you!
I really appreciate your help
You’ve been great to me. Thank you!
Thank you for your articles. They are very helpful to me. Can you help me with something?
I must appreciate the way you have expressed your feelings through your blog!
Heya i am for the first time here. I came across this board and I find It truly useful & it helped me out much. I hope to give something back and aid others like you helped me.
There is certainly a lot to learn about this topic. I love all the points you made.
I really enjoy the post.Much thanks again. Cool.
Great article post.Really looking forward to read more. Really Cool.
Kudos! I like this!descriptive essay writing write my essay phd writers
I loved your post.Really looking forward to read more. Really Cool.
I could not refrain from commenting. Perfectly written!
I have been exploring for a bit for any high quality articles or blog posts on this kind of house .
Exploring in Yahoo I finally stumbled upon this website. Studying this
information So i am happy to express that I have an incredibly
good uncanny feeling I came upon just what I needed.
I such a lot without a doubt will make certain to don?t
overlook this site and provides it a look regularly.
I seriously love your blog.. Very nice colors & theme. Did you create this website yourself?
Please reply back as I’m trying to create my very own blog and would love to know where you got this
from or just what the theme is named. Thank you!
Hey there just wanted to give you a quick heads up.
The text in your content seem to be running off
the screen in Ie. I’m not sure if this is a format issue or something to do
with internet browser compatibility but I thought I’d post to let you know.
The design look great though! Hope you get the problem fixed
soon. Many thanks
Link exchange is nothing else but it is just placing the other person’s blog link on your page at suitable place and other person will also do same in favor of you.
Hey I know this is off topic but I was wondering if you knew of any
widgets I could add to my blog that automatically tweet my newest twitter updates.
I’ve been looking for a plug-in like this for quite some time and was hoping maybe
you would have some experience with something like this.
Please let me know if you run into anything.
I truly enjoy reading your blog and I look forward to your
new updates.
This is the perfect website for anybody who really wants to find out about this topic.
You know a whole lot its almost hard to argue with you
(not that I actually would want to…HaHa). You certainly put a brand
new spin on a subject that has been discussed for decades.
Wonderful stuff, just excellent!
Thanks for sharing your thoughts on website. Regards
An interesting discussion is definitely worth comment.
I do believe that you ought to write more about this subject matter, it may not be
a taboo matter but usually folks don’t talk about such issues.
To the next! Cheers!!
I go to see everyday a few blogs and blogs to read content, but this weblog
presents quality based posts.
Everything is very open with a clear clarification of the issues.
It was really informative. Your website is useful.
Thanks for sharing!
An interesting discussion is definitely worth comment.
I believe that you should publish more on this topic,
it may not be a taboo matter but typically people don’t speak about such topics.
To the next! Cheers!!